Category
Programming
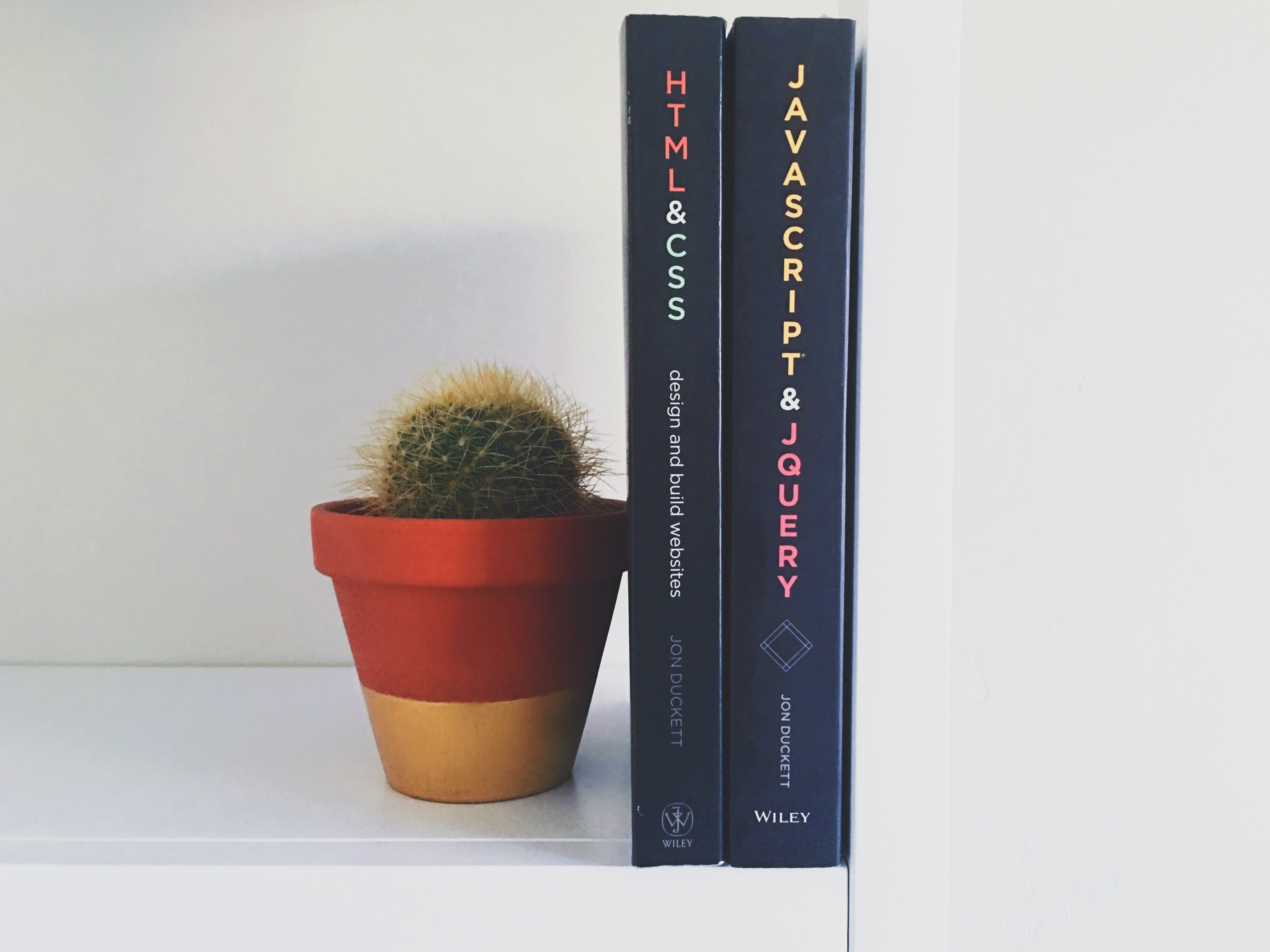
Description
Along with HTML and CSS, the computer language known as JavaScript, or JS, is one of the foundational elements of the World Wide Web. 98% of websites will utilize JavaScript on the client side by the year 2022 to control webpage functionality, frequently integrating third-party libraries.
The development and history of the internet will also be covered.
Course Content
8 MODULEs • 3 months total length
A Brief Introduction to JavaScript • Linking a JavaScript File • Values and Variables • Practice Assignments •
Data Types • let, const and var • Basic Operators •
Operator Precedence • Strings and Template Literals • if / else Statements • Type Conversion and Coercion • Truthy and Falsy Values • Equality Operators: == vs. === • Boolean Logic • Logical Operators • The switch Statement • Statements and Expressions • The Conditional (Ternary) Operator
Activating Strict Mode • Functions • Function Declarations vs. Expressions • Arrow Functions • Functions Calling Other Functions • Introduction to arrays • Basic Array Operations (Methods) • Introduction to Objects • Dot vs. Bracket Notation • Object Methods •
Iteration: The for Loop • Looping Arrays, Breaking and Continuing • Looping Backwards and Loops in Loops •
The while Loop
What's the DOM and DOM Manipulation • Selecting and Manipulating Elements • Handling Click Events • Manipulating CSS Styles • Working With Classes
Destructuring Arrays • Destructuring Objects • The Spread Operator (...) • Rest Pattern and Parameters • Short Circuiting (&& and ||) • The Nullish Coalescing Operator (??) • Logical Assignment Operators • Looping Arrays: The for-of Loop • Enhanced Object Literals • Optional Chaining (?.) • Looping Objects: Object Keys, Values, and Entries • Maps: Fundamentals • Maps: Iteration • Working With Strings - Part 1 • Working With Strings - Part 2 • Working With Strings - Part 3
Simple Array Methods • The new at Method • Looping Arrays: forEach • forEach With Maps and Sets • LoopingCreating DOM Elements • Data Transformations: map, filter, reduce • The map Method • The filter Method • The reduce Method • Sorting Arrays
Selecting, Creating, and Deleting Elements • Styles, Attributes and Classes • Implementing Smooth Scrolling • Types of Events and Event Handlers • Event Propagation: Bubbling and Capturing • Event Delegation: Implementing Page Navigation • DOM Traversing • Passing Arguments to Event Handlers • Implementing a Sticky Navigation: The Scroll Event • Revealing Elements on Scroll • Lazy Loading Images • Building a Slider Component • Lifecycle DOM Events • Efficient Script Loading: defer and async
What is Object-Oriented Programming? • OOP in JavaScript • Constructor Functions and the new Operator • Prototypes • Prototypal Inheritance on Built-In Objects • ES6 Classes • Setters and Getters • Static Methods • Object.create • Inheritance Between "Classes": Constructor Functions • Inheritance Between "Classes": ES6 Classes • Inheritance Between "Classes": Object.create • Encapsulation: Protected Properties and Methods • Encapsulation: Private Class Fields and Methods • Chaining Methods
Asynchronous JavaScript, AJAX and APIs • IMPORTANT: API URL Change • Our First AJAX Call: XMLHttpRequest • Promises and the Fetch API • Consuming Promises • Handling Rejected Promises • Throwing Errors Manually • Asynchronous Behind the Scenes: The Event Loop • The Event Loop in Practice • Promisifying the Geolocation API • Consuming Promises with Async/Await • Error Handling With try...catch • Returning Values from Async Functions • Running Promises in Parallel